In Python, there is a cool module called "types" that defines the names of the different types of variables. We can explore this module to see how Python recognizes a variable type without it being declared by the programmer.
Identifying a datatype in Python takes a very small amount of code; this hearkens back to my previous blog post that talk about the conciseness of Python's language in one-liners of code. Because Python is dynamically typed, the "type" module is able to recognize any valid datatype for what it it.
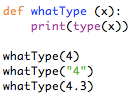
An here is the output:
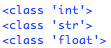
Dictionaries:
Now I will briefly introduce a datatype you may have not seen before. "A Python dictionary is a mapping of unique keys to values." Dictionaries sort of act like two lists joined together or a 2D array, where there is an association between the two parts. I am introducing dictionaries, not only because they are an interesting and useful datatype to know about and one I have not encountered before in other languages, but because I will elaborate on the above example where we recognize the datatypes of each variable to show that even more complex datatypes are recognized.
Here is the example again, with an example for a dictionary as well as a list included:
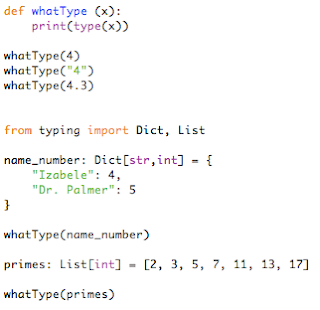
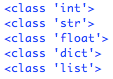
Other than the possibly unfamiliar syntax of a dictionary, you might notice something else unfamiliar here. In this example, I declared name_number as a dictionary and primes as a list. Since Python is dynamically typed, I did not need to do this, but I wanted to demonstrate that it is still possible and could be useful to do, especially as your programs get more complicated.
I could have just as easily done the following:
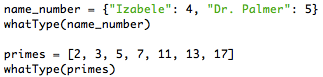
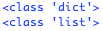
Sources:
http://www.secnetix.de/olli/Python/dynamic_typing.hawk
https://medium.com/@ageitgey/learn-how-to-use-static-type-checking-in-python-3-6-in-10-minutes-12c86d72677b
http://www.pythonforbeginners.com/dictionary/how-to-use-dictionaries-in-python
http://developer.rhino3d.com/guides/rhinopython/python-datatypes/#tuple
Explore this last link to read about tuples, another datatype in Python. Tuples look and act very much like lists, but there are some important differences. I included this reference as supplemental reading as I feel it is important information, but does not necessarily relate to this post.